Intro to React Native continued…
Components
Ok so in my last blog we got a react native app up and running, if you're reading this before that one head on over HERE and give that a read first, I’ll wait…
Alright I’m gonna talk about react native components. To create a component we need to import some libraries.
First, at the top of your text editor page we need to import React from ‘react’ then on the next line we need to import some stuff from the react-native library for now we will import { Text, StyleSheet, View } from ‘react-native’ that should all look like this…

So here we are importing the entire React library first then, because we don’t need to whole react-native library we import specific variables as shown above. These three are the most common for getting started. We won't be using View in this post but it will be explained in the next one (cliffhanger…)
Now we can create a component ( a function that returns some ‘JSX’ ).

So here is a basic component that will render Hello World! to the screen. I have used an arrow function just because I like how it looks. you could also use a keyword function like this:
const ComponentsScreen = function() {}
both work the very same. The return is where we will tell react native what we want to show on the screen of the device. Here we have the <Text></Text> tag with some words written in between them, so Hello World! then shows up on the device’s screen:
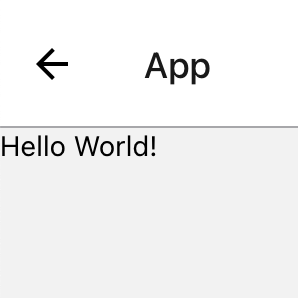
Now that we have something on the screen it would be nice to be able to style it. We can now use the Stylesheet variable that we imported earlier. Lets create a stylesheet so we can change how the text appears on the screen, to do this we type the following:
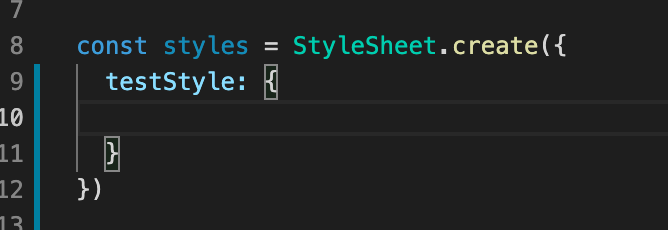
so textStyle is where we will define the properties we want to style for the Text element. Now just because I called this textStyle does not mean it will automatically style the Text element they have to be connected somehow. I will add a property of fontSize and let's make it 30 for now.

Okay, now lets assign it to the Text that we want to style:

I will explain how this all works in my next post but for now you can see that we added style inside the opening <Text> tag. To finish off this component file we need to export it so it can be used elsewhere:

below is a full view of a very basic component file:

This is basically the boilerplate code of a component in react native so get used to typing it out, or you could make a custom snippet.
To finish off this post I will show you how to make a custom snippet in VSCode, snippets are very helpful as they save so much time and you can focus on more important tasks without needing to worry about the boilerplate stuff. Okay so really quickly, this is how to make a custom snippet in VSCode:
In VSCode on a Mac go to Code at the top of your screen then select preferences then user snippets (on a windows machine it’s File instead of Code). This is where you will create your snippet.
Don’t forget to select your language, for this it’s JS…

The best way to get the syntax correct is to go to snippet generator HERE and copy and paste your code.

On the left is what you pasted and on the right, it is formatted for VSCode, you can also format it for Sublime text or Atom. On the top left add a description and a prefix. The prefix is what you will type to trigger the snippet I chose imp so typing imp then hitting the tab key will generate all this code. Cool right!? so just click on Copy snippet on the bottom right, go back to your text editor and paste that all in like so:

and there you have it! you can also add placeholders for you to then type the few different words you may always need to change so instead of having the same text every time you can do this:

Notice I placed $1 after const so I can first name my component(which will be different every time) $2 in the <Text></Text> tags and then $3 after the export default ( just hit the tab key to go through each one ) so that I can get up and running so much faster than typing this from scratch every time. I really would suggest creating your own snippets and also looking into snippets in general, I find them very helpful.
So that’s it for this post as promised I will continue with the react native series so stay tuned to continue learning along with me. Thanks for reading!